Recently, I was building a GitHub Actions pipeline, and needed to access output from Terraform. There’s a very convenient feature in Terraform that allows you to get output from your applied .tf files. You can call those values from the shell directly, and assign them to variables in your shell.
What you need to do to define an output, and then call it from your shell is the following=:
output "rgname" {
value = azurerm_resource_group.aks-sd-rg.name
}
Which you can then call from a shell like this:
terraform output rgname
And if you want to assign this to a local variable, you can do this:
rgname=$(terraform output rgname)
However, when using this in a GitHub Action, this fails consistently. This can very easily be fixed in the setup of the Terraform action in your GitHub action:
- name: Setup Terraform
uses: hashicorp/setup-terraform@v1
with:
terraform_wrapper: false
And with that you should be able to refer to the output correctly. Let me show you a working example of this on GitHub.
Minimal PoC on GitHub
I built a very minimal PoC of this on GitHub. A very small TF file is used to generate output:
locals {
test = "this is the output"
}
output test{
value = local.test
}
And I have two actions. One without the terraform_wrapper
, one with the terraform_wrapper
. In each action, I execute the following small shell script at the end:
output=$(terraform output test)
echo $output
Without the wrapper, this is the result of the Action:
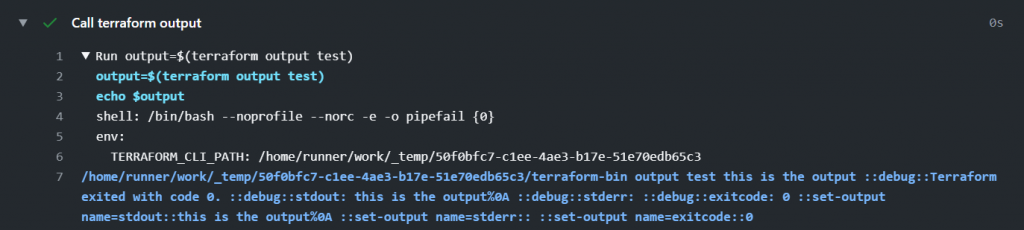
With the wrapper in place, this is the result of the Action:
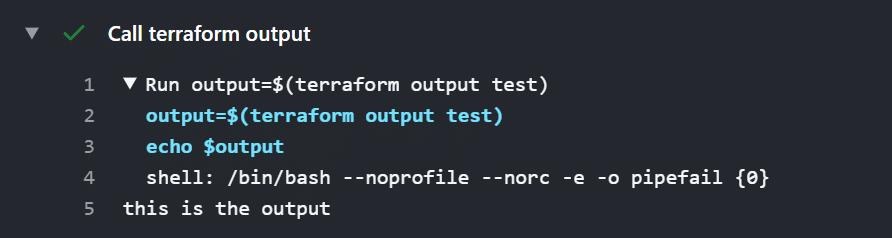
You can check out the full actions files I used for this here:
Summary
If you need to call terraform output in a GitHub action, make sure to include terraform_wrapper: false
in your GitHub actions file. This took me a couple hours to figure out, so I hope to save you those hours.